Create a CRUD Application with Laravel 5.2 part-5
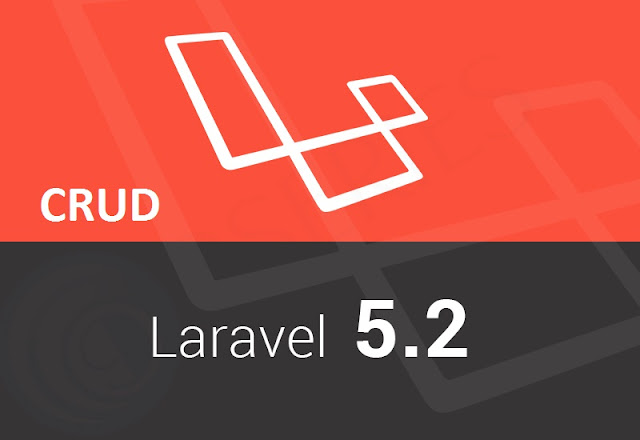
Now as we have listed our users, let’s write the code for creating new users. To create a new user, we will need to create the Controller method and bind the view for displaying the new user form. We would need to create the Controller method for saving the user too. Now as we have bound the resourceful Controller, we don’t need to create separate routes for each of our request. Laravel will handle that part if we use REST methods.
So first let’s edit the controller at /app/http/controllers/UsersController.php to add a method for displaying the view:
public function create()
{
return View::make('users.create');
}
This will call a view at /app/resources/users/create.blade.php. So let’s define our create.blade.php view as follows:
@extends('layouts.users')
@section('main')
<h1>Create User</h1>
{{ Form::open(array('route' => 'users.store')) }}
<ul>
<li>
{{ Form::label('name', 'Name:') }}
{{ Form::text('name') }}
</li>
<li>
{{ Form::label('username', 'Username:') }}
{{ Form::text('username') }}
</li>
<li>
{{ Form::label('password', 'Password:') }}
{{ Form::password('password') }}
</li>
<li>
{{ Form::label('password', 'Confirm Password:') }}
{{ Form::password('password_confirmation') }}
</li>
<li>
{{ Form::label('email', 'Email:') }}
{{ Form::text('email') }}
</li>
<li>
{{ Form::label('phone', 'Phone:') }}
{{ Form::text('phone') }}
</li>
<li>
{{ Form::submit('Submit', array('class' => 'btn')) }}
</li>
</ul>
{{ Form::close() }}
@if ($errors->any())
<ul>
{{ implode('', $errors->all('<li class="error">:message</li>')) }}
</ul>
@endif
@stop
Let’s try to understand our preceding view. Here we are extending the users layout we created in our List Users section. Now in the main section, we are using Laravel’s Form helper to generate our form. This helper generates HTML code via its methods such as label, text, and submit.
Refer to the following code:
{{ Form::open(array('route' => 'users.store')) }}
The preceding code will generate the following HTML code:
<form method="POST" action="http://localhost/users" accept-charset="UTF-8">