Create a CRUD Application with Laravel 5.2 part-6
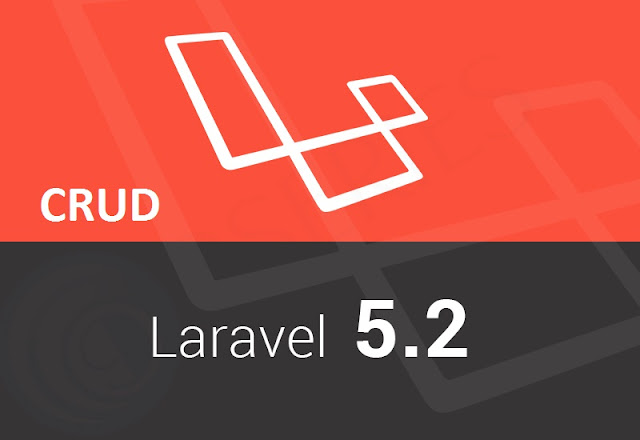
public function store()
{
$input = Input::all();
$validation = Validator::make($input, User::$rules);
if ($validation->passes())
{
User::create($input);
return Redirect::route('users.index');
}
return Redirect::route('users.create')
->withInput()
->withErrors($validation)
->with('message', 'There were validation errors.');
}
Here we are first validating all the input that came from the user. The Input::all () function fetches all the $_GET and $_POST variables and puts it into a single array. The reason why we are creating the single input array is so we can check that array against validation rules’ array. Laravel provides a very simple Validation class that can be used to check validations. We could use it to check whether validations provided in the rules array are followed by the input array by using the following line of code:
$validation = Validator::make ($input, User::$rules);
Rules can be defined in an array with validation attributes separated by the column “|”. Here we are using User::$rules where User is our Model and it will have following code:
class User extends Eloquent {
protected $guarded = array('id');
protected $fillable = array('name', 'email');
public static $rules = array(
'name' => 'required|min:5',
'email' => 'required|email'
);
}
As you can observe we have defined two rules mainly for name and e-mail input fields. If you are wondering about $guarded and $fillable variables, these variables are used to prevent mass assignment. When you pass an array into your Model’s create and update methods, Laravel tries to match the right columns and sets values in the database. Now for instance, if a malicious user sends a hidden input named id and changes his ID via the update method of your form, it could be a huge security hole; to prevent this, we should define the $guarded and $fillable arrays. The $guarded array will guard the columns defined in the guarded array, that is, it will prevent anyone from changing values in that column. The $fillable array will only allow elements defined in $fillable to be updated.
READ 20 Best Podcasts For Web Developers
Now we can use the $validation instance we created to check for validations.
$result = $validation->passes();
echo $result; // True or false
If you see our code now, we are checking for validation via the passes() method in our Store() method of UserController. Now if validation gets passed, we can use our user Model to store data into our database. All you need to do is call the Create method of the Model class with the $input array. So refer to the following code:
User::create($input);
The preceding code will store our $input array into the database; yes, it’s equivalent to your SQL query.
Insert into user(name,password,email,city) values (x,x,..,x);
Here we have to fill either the $fillable or $guarded array in the model, otherwise, Laravel will throw a mass assignment exception. Laravel’s Eloquent object automatically matches our input array with the database and creates a query based on our input array. Don’t you think this is a simple way to store input into the database? If user data is inserted, we are using Laravel’s redirect method to redirect it to our list of users’ pages. If validation fails, we are sending all of the input with errors from the validation object into our create users form.