Create a CRUD Application with Laravel 5.2 part-1
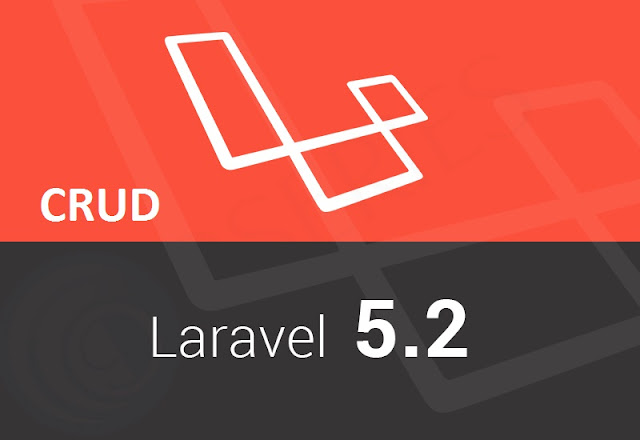
- List users (read users from the database)
- Create new users
- Edit user information
- Delete user information
- Adding pagination to the list of users
http://localhost/phpmyadmin
;
if you don’t have phpMyAdmin installed, use the MySQL admin tool
workbench to connect with your database and create a new database.Requirements -> html form –> please install this and follow instructions from official site
Preview

Now we need to configure Laravel to connect with our database. So head over to your Laravel application folder, open
config/database.php
, change the MySQL array, and match your current database settings. Here is the MySQL database array from database.php
file:'mysql' => array( 'driver' => 'mysql', 'host' => 'localhost', 'database' => '<yourdbname>', 'username' => 'root', 'password' => '<yourmysqlpassord>', 'charset' => 'utf8', 'collation' => 'utf8_unicode_ci', 'prefix' => '', ),Now we are ready to work with the database in our application. Let’s first create the database table
Users
via the following SQL queries from phpMyAdmin or any MySQL database admin tool;CREATE TABLE IF NOT EXISTS 'users' ( 'id' int(10) unsigned NOT NULL AUTO_INCREMENT, 'username' varchar(255) COLLATE utf8_unicode_ci NOT NULL, 'password' varchar(255) COLLATE utf8_unicode_ci NOT NULL, 'email' varchar(255) COLLATE utf8_unicode_ci NOT NULL, 'phone' varchar(255) COLLATE utf8_unicode_ci NOT NULL, 'name' varchar(255) COLLATE utf8_unicode_ci NOT NULL, 'created_at' timestamp NOT NULL DEFAULT '0000-00-00 00:00:00', 'updated_at' timestamp NOT NULL DEFAULT '0000-00-00 00:00:00', PRIMARY KEY ('id') ) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci AUTO_INCREMENT=3 ;Now let’s seed some data into the
Users
table so when we fetch the users we won’t get empty results. Run the following queries into your database admin tool:INSERT INTO 'users' ('id', 'username', 'password', 'email', 'phone', 'name', 'created_at', 'updated_at') VALUES (1, 'john', 'johndoe', 'johndoe@gmail.com', '123456', 'John', '2013-06-07 08:13:28', '2013-06-07 08:13:28'), (2, 'amy', 'amy.deg', 'amy@outlook.com', '1234567', 'amy', '2013-06-07 08:14:49', '2013-06-07 08:14:49');
Note
Later we will see how we can manage our database via laravel 5.2’s powerful migrations features. At the end of this chapter, I will introduce you to why it’s not a good practice to manually create SQL queries and make changes to the database structure. And I know that passwords should not be plain too!Listing the users – read users from database
Let’s read users from the database. We would need to follow the steps described to read users from database:- A route that will lead to our page
- A controller that will handle our method
- The Eloquent Model that will connect to the database
- A view that will display our records in the template
/app/http/routes.php
. Add the following line to the routes.php
file:Route::resource('users', 'UserController');If you have noticed previously, we had
Route::get
for displaying our page Controller. But now we are using resource
. So what’s the difference?In general we face two types of requests during web projects: GET and POST. We generally use these HTTP request types to manipulate our pages, that is, you will check whether the page has any POST variables set; if not, you will display the user form to enter data. As a user submits the form, it will send a POST request as we generally define the
<form method="post">
tag in our pages. Now based on page’s request type, we set the code to
perform actions such as inserting user data into our database or
filtering records.What Laravel provides us is that we can simply tap into either a GET or POST request via routes and send it to the appropriate method. Here is an example for that:
Route::get('/register', 'UserController@showUserRegistration'); Route::post('/register', 'UserController@saveUser');See the difference here is we are registering the same URL,
/register
, but we are defining its GET method so Laravel can call UserController
class’ showUserRegistration
method. If it’s the POST method, Laravel should call the saveUser
method of the UserController
class.routes.php
file and guess which Controller and which method of Controller handles
the part you are interested in, developing it further or solving some
bug. Even some other developer who is not used to your project will be
able to understand how things work and can easily help move your
project. This is because he would be able to somewhat understand the
structure of your application by checking routes.php
.Now imagine the routes you will need for editing, deleting, or displaying a user. Resource Controller will save you from this trouble. A single line of route will map multiple restful actions with our resource Controller. It will automatically map the following actions with HTTP verbs:
HTTP VERB | ACTION |
---|---|
GET | READ |
POST | CREATE |
PUT | UPDATE |
DELETE | DELETE |