Create a CRUD Application with Laravel 5.2 part-2
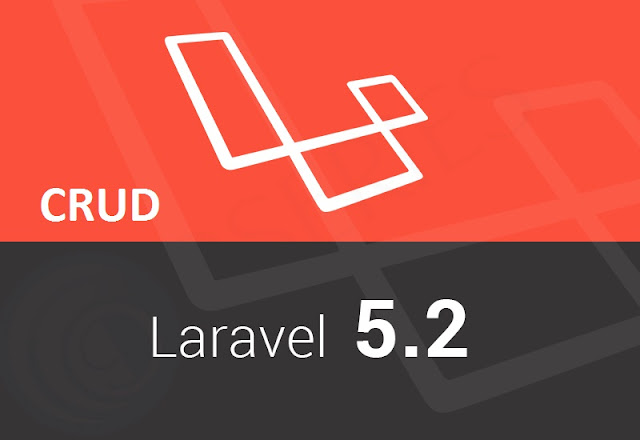
$ php artisan make:controller usercontroller
This will generate UsersController.php with all the RESTful empty methods, so you will have an empty structure to play with. Here is what we will have after the preceding command:
class UserController extends BaseController {
/**
* Display a listing of the resource.
*
* @return Response
*/
public function index()
{
//
}
/**
* Show the form for creating a new resource.
*
* @return Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @return Response
*/
public function store()
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param int $id
* @return Response
*/
public function update($id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return Response
*/
public function destroy($id)
{
//
}
}
Now let’s try to understand what our single line route declaration created relationship with our generated Controller.
HTTP VERB Path Controller Action/method
GET /Users Index
GET /Users/create Create
POST /Users Store
GET /Users/{id} Show (individual record)
GET /Users/{id}/edit Edit
PUT /Users/{id} Update
DELETE /Users/{id} Destroy