Create a CRUD Application with Laravel 5.2 part-3
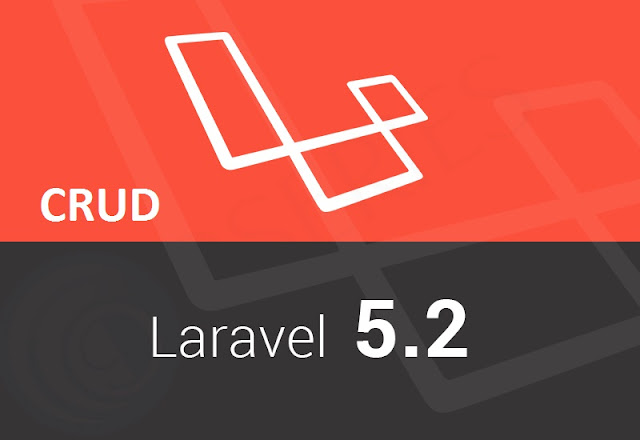
$ php artisan routes
Now let’s get back to our basic task, that is, reading users. Well now we know that we have UserController.php at /app/controller with the index method, which will be executed when somebody launches http://localhost/laravel/public/users. So let’s edit the Controller file to fetch data from the database.
Well as you might remember, we will need a Model to do that. But how do we define one and what’s the use of Models? You might be wondering, can’t we just run the queries? Well Laravel does support queries through the DB class, but Laravel also has Eloquent that gives us our table as a database object, and what’s great about object is that we can play around with its methods. So let’s create a Model.
If you check your path /app/User.php, you will already have a user Model defined. It’s there because Laravel provides us with some basic user authentication. Generally you can create your Model using the following code:
class User extends Eloquent {}
Now in your controller you can fetch the user object using the following code:
$users = User::all();
$users->toarray();
Yeah! It’s that simple. No database connection! No queries! Isn’t it magic? It’s the simplicity of Eloquent objects that many people like in Laravel.
READ Understanding Design Patterns in Laravel
But you have the following questions, right?
How does Model know which table to fetch?
How does Controller know what is a user?
How does the fetching of user records work? We don’t have all the methods in the User class, so how did it work?
Well models in Laravel use a lowercase, plural name of the class as the table name unless another name is explicitly specified. So in our case, User was converted to a lowercase user and used as a table to bind with the User class.
Models are automatically loaded by Laravel, so you don’t have to include the reference of the Model file. Each Model inherits an Eloquent instance that resolves methods defined in the model.php file at vendor/Laravel/framework/src/Illumininate/Database/Eloquent/ like all, insert, update, delete and our user class inherit those methods and as a result of this, we can fetch records via User::all().
So now let’s try to fetch users from our database via the Eloquent object. I am updating the index method in our app/controllers/UsersController.php as it’s the method responsible as per the REST convention we are using via resource Controller.
public function index()
{
$users = User::all();
return View::make('users.index', compact('users'));
}